Datetimes#
Lesson Content
Dates
Datetimes
Timedeltas
Context#
Dates and times are a common part of working with scientific data. They are very common fields. While dates and times are commonly represented as strings, you can also represent them using Python-specific objects that come with added functionality specific to dates and times.
Python library datetime
#
The datetime
library has three major components:
date()
- for handling a date (Ex. 2021-06-28)datetime()
- for handling a specific time of a particular date (Ex. 2021-06-28 at 12:31pm)timedelta()
- for handling a length of time (Ex. 1 hour, 2 weeks, 3.5 years)
Dates#
from datetime import date
date(2017, 6, 30)
datetime.date(2017, 6, 30)
# assigning a date to a variable
birthday = date(2019, 9, 12)
Why bother?#
The dates are nice, but why put in the effort? Your data by default probably uses a string, for example 2016-06-30
, so why not stick with that?
Reason 1#
If Python knows it’s a date it can help you find errors.
date(2017, 6, 31)
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
Cell In[4], line 1
----> 1 date(2017, 6, 31)
ValueError: day is out of range for month
# Feb 29 exists during a leap year
date(2012, 2, 29)
datetime.date(2012, 2, 29)
# But not during other years!
date(2011, 2, 29)
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
Cell In[6], line 2
1 # But not during other years!
----> 2 date(2011, 2, 29)
ValueError: day is out of range for month
Reason 2#
Added organization through quick accessing of elements.
We’ll talk about this more below.
Accessing parts of a date#
Once you have a date created you can easily pull out the piece you want.
campaign_start = date(2011, 5, 15)
campaign_start.month
5
# Monday is 0 and Sunday is 6
campaign_start.weekday()
6
📝 Check your understanding
Create a date object that represents your birthday.
Using dates()
with other objects#
You can do what you want with these objects, such as putting them in lists or dictionaries.
holidays = [date(2021, 1, 1), date(2021, 1, 18), date(2021, 11, 25)]
holidays_dict = {
'New Years Day': date(2021, 1, 1),
'MLK Day': date(2021, 1, 18),
'Thanksgiving': date(2021, 11, 25),
}
holidays_dict['Thanksgiving']
datetime.date(2021, 11, 25)
A comment on calendars#
Turns out there are actually lots of different calendars that can be used to track time. The one you are most likely familiar with is the Gregorian calendar (using years, months, and days). Another common one that you will use is a Julian calendar, which starts on January 1st and counts the days one by one.
If you use model data you will also find more types of calendars, such as 365_day
(just ignores leap days) or 360_day
(every month has 30 days).
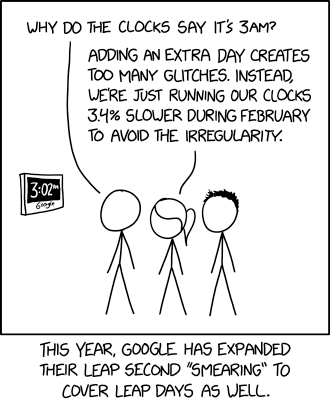
📝 Check your understanding
holidays_dict = {
'New Years Day': date(2021, 1, 1),
'MLK Day': date(2021, 1, 18),
'Thanksgiving': date(2021, 11, 25),
}
What is the output of holidays_dict['MLK Day'].day
?
Datetimes#
Creating datetime()
objects manually#
from datetime import datetime
# Created just like `date` objects
datetime(2021, 9, 21, 13, 45, 0)
datetime.datetime(2021, 9, 21, 13, 45)
We have the same ability as with date()
s to access the different parts of the datetime object. One place this could come in handy is if we need to narrow down our data by some aspect of the date.
example = datetime(2021, 9, 21, 13, 45, 0)
if example.hour >= 12 and example.hour <= 18:
print('sample taken in the afternoon')
sample taken in the afternoon
A comment on timezones#
The datetime
library also has the ability to keep track and convert timezones. Timezones can cause a lot of chaos in the data world. We aren’t going to talk about them in depth but know that if you need to track timezones for whatever reason datetime
can handle that.
Creating date()
s and datetime()
s from strings#
Thus far we have created our time objects by inputing the integer number of the year, month, etc. Much more commonly, though, you aren’t creating them from scratch, you are creating them from a string. For that we have strptime
and strftime
.
Syntax |
Description |
---|---|
|
Convert from string to datetime |
|
Convert from datetime to string |
All of the following strings can be converted programatically into datetime objects:
'2019-01-29'
'01-31-88'
'September 12 7:00PM'
'Apr 11 2001 13:00:00'
'210 9:00'
And so many other combinations
The key part of converting from string to datetime and vice versa is that we need to tell the computer what is supposed to be looking for in the string. For that we have labels, or directives, such as %Y
or %d
. The place to consult for format strings is strftime.org.
Some Examples#
Example 1#
date_string = '2019-01-29'
Example 2#
date_string = 'Apr 11 2001 13:00:00'
Example 3#
Switching date formats
start_date_string = '2016234'
# Goal: '2016-08-21 00:00:00'
📝 Check your understanding
What is the format string corresponding to the following datetime string?
'8/30/2019 22:13:00'
, which shows the date August 30th 2019 at 10:13PM (military time).
timedelta()
objects#
While date()
sand datetime()
s represent a specific moment in time, timedelta()
s represent a length of time, for example, one week, two hours, or 15 seconds. They aren’t connected to any particular moment, they just represent a set length of time.
from datetime import timedelta
# Representing 3 days
timedelta(days=3)
datetime.timedelta(days=3)
date.today() + timedelta(days=3)
datetime.date(2023, 8, 6)
# Negatives also work
date.today() + timedelta(days=-3)
datetime.date(2023, 7, 31)
When we combine timedelta()
s and datetime()
s what we get is the ability to really quickly determine how long there is between two things.
# Combine lengths of time
datetime.now() + timedelta(days=3, hours=1)
datetime.datetime(2023, 8, 6, 17, 31, 38, 601208)
# If you want to find the difference between two lengths of time
date.today() - date(2021, 11, 25)
datetime.timedelta(days=616)
📝 Check your understanding
How many days are there between today and Thanksgiving of this year (November 25th 2022)?
Code Summary#
from datetime import datetime, date, timedelta
# Creating a single date
independence_day = date(2022, 7, 4)
# Creating a single datetime
flight_start = datetime(2021, 12, 17, 9, 28, 00)
# Inspecting dates/datetimes
print('independence day was on the day of week :', independence_day.weekday()) # 0 is Monday
print('flight month:', flight_start.month)
# Parsing strings
date_string = '2019-01-29'
dt = datetime.strptime(date_string, '%Y-%m-%d')
print("I'm a datetime object:", dt)
# Timedeltas
timedelta(days=7)
print('one week from today will be:', datetime.today()+timedelta(days=7))
print('this year started ', datetime.today() - datetime(2022, 1, 1), 'ago')
independence day was on the day of week : 0
flight month: 12
I'm a datetime object: 2019-01-29 00:00:00
one week from today will be: 2023-08-10 16:31:38.619370
this year started 579 days, 16:31:38.619419 ago