Variables, Data Types and If statements Answers#
Part 1#
Assigning Variables#
Create a variable called
location
and assign it the value: Quito, Ecuador. Print your variable.
location = 'Quito, Ecuador'
# or
location = "Quito, Ecuador"
print(location)
Quito, Ecuador
Instead of print(location)
you may have used quotes, as shown below.
print('location')
location
When you run that cell you’ll notice that it prints the word location, because you gave print
the string value location. When you remove the quotes the print gives the value of the variable.
Create two variables that are numbers and print the result of one of them divided by the other.
number1 = 45
number2 = 5
print(number1/number2)
9.0
What do you expect to be the output of the following block of code?
my_fav_icecream = 'mint'
your_fav_icecream = 'blue moon'
my_fav_icecream = 'peanut butter cup'
print(my_fav_icecream)
# peanut butter cup
Data Types#
What are the data types of each of the following values?
True
– Boolean832
– integer0.001
– float'giant kelp'
– string"false"
– string"5"
– string
Check that you are correct using the type()
function, as shown below.
type('cheese')
str
Booleans#
What is a boolean? When might a boolean be useful?
# Booleans are a data type that have only two possible values -- either 0 or 1.
# Booleans are often useful when doing comparisons.
The following cell represents some data you are working with.
cloud_cover = 15
city = 'Oshkosh'
cfc_present = True
ozone = 3.8
Run the cell above, and use the variables to individually check for each of the following conditions:
cloud cover is less than 90
city is not Minneapolis
there are no cfcs present
ozone is greater than or equal to 2
cloud_cover < 90
True
city != 'Minneapolis'
True
cfc_present == False
False
ozone >= 2
True
Explain the difference between
=
and==
.
# A single = is used to assign a value to a variable, while a double == is "equals to" and is a comparison
# The result of == is a Boolean output - either True or False. There isn't usually output when using a single =, just a new variable.
Logical Operators#
How is
and
different fromor
?
# and checks if both statements are true, while or checks if any single one of them is true.
Fill out the values for
p and q
andp or q
in the following table, given each combination of the possible values forp
and forq
.
p |
q |
p and q |
p or q |
---|---|---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
For each of the following scenarios do you think it would make more sense to use
and
oror
?
You have several images and you want to make sure that at least one of them has the wavelength you need. – or
You have an algorithm which requires three variables in a specific range and you want to check those three variables in your image to make sure the image will work. – and
In a particular dataset you are looking at invalid results have the value -999. You also know that values above 1000 are not suitable for your use case. You need to check the dataset to make sure that neither of those things are true. – and
Write a statement using the following operators:
and
,!=
visibility = 62
visibility > 40 and visibility != 74
True
Write a statement using the following operators:
or
,+
speed = 12
speed + 10 > 15 or speed + 25 > 15
True
If statements#
Write an if statement that uses the following elements:
if
,<=
,or
depth = 200
if depth <= 150 or depth < 500:
print('ideal conditions')
ideal conditions
You have species data with the variable
family
and you would like to assign the species to a group based on the family. The groups are:
Group A -
pinaceae
,lamiaceae
Group B -
aceraceae
,salicaceae
Write a statement that checks the family
variable and informs the user if the species belongs in group A or group B.
family = 'salicaceae'
if family == 'pinaceae' or family == 'lamiacae':
print('species in group A')
elif family in family == 'aceraceae' or family == 'salicaceae':
print('species in group B')
else:
print('no family found.')
species in group B
Practice Problems Part 2#
Question 1#
Write some code to take the mean value of the three sun angles below.
sun_angle1 = 45
sun_angle2 = 32
sun_angle3 = 67
# Take the mean
# Output the mean to the user
mean_angle = (sun_angle1 + sun_angle2 + sun_angle3)/3
print(mean_angle)
48.0
Question 2#
A) Google “Python operators”. Find a webpage with some operators and use two of them below. If you are having a hard time finding a webpage you can use this one, which is the first page that shows up when I run the search.
# Floor division
10 // 3
3
# Exponents
3**4
81
B) Fill in the # Your code here
space below to compute the area of a circle given the radius. You can approximate the value of pi with 3.14
.
radius = 4
# Your code here
area = 3.14 * radius**2
print(area)
50.24
Hint I haven’t told you how to take the square of a variable, so you will either have to do that using the tools you have or do a google search for “square of a number Python”.
C) Calculate 2 to the 8th power. Assign it to a variable and print the output. Include a string in the print statement that says: 2 to the 8th power is:
output = 2**8
print('2 to the 8th power is: ', output)
2 to the 8th power is: 256
Question 3#
What is the output of the following block of code?
updated_temp = 72
print(updated_temp)
updated_temp = updated_temp + 5
updated_temp = updated_temp + 2
updated_temp = updated_temp -9
print(updated_temp)
# 72
# 70
Question 4#
Use a comparison (less than, equal to, etc.) to determine if the result of 3 to the power of some number (the input variable exponent
) is between 100 and 1000.
exponent = 7
3**exponent > 100 and 3**exponent < 1000
False
Question 4#
cloud_cover = 15
city = 'Oshkosh'
cfc_present = True
ozone = 3.8
A) Use the variables in the cell above and check that the following conditions are true:
Cloud cover is less than 90
city is not Minneapolis
There are no cfcs present
ozone is greater than or equal to 2 and above 0.5
Print a message to the user informing them if all of the conditions were true or not.
I realize on this question that ozone being greater than 2 and above 0.5 is repetitive, so my apologies for the nonsensical request. In the solution I just dropped the “above 0.5” off in my solution
if cloud_cover < 90 and city != 'Minneapolis' and cfc_present == False and ozone >= 2:
print('all conditions met')
else:
print('not all conditions met')
not all conditions met
B) Using the same variables and conditions as in part A, change your code so that it checks if any of the conditions are true.
if cloud_cover < 90 or city != 'Minneapolis' or cfc_present == False or ozone >= 2:
print('at least one condition met')
at least one condition met
Question 5#
As a variable, wind is often expressed as two different numbers, which together make up the complete wind vector:
wind speed
wind direction
Wind speed is expressed in degrees and follows the following quadrant:
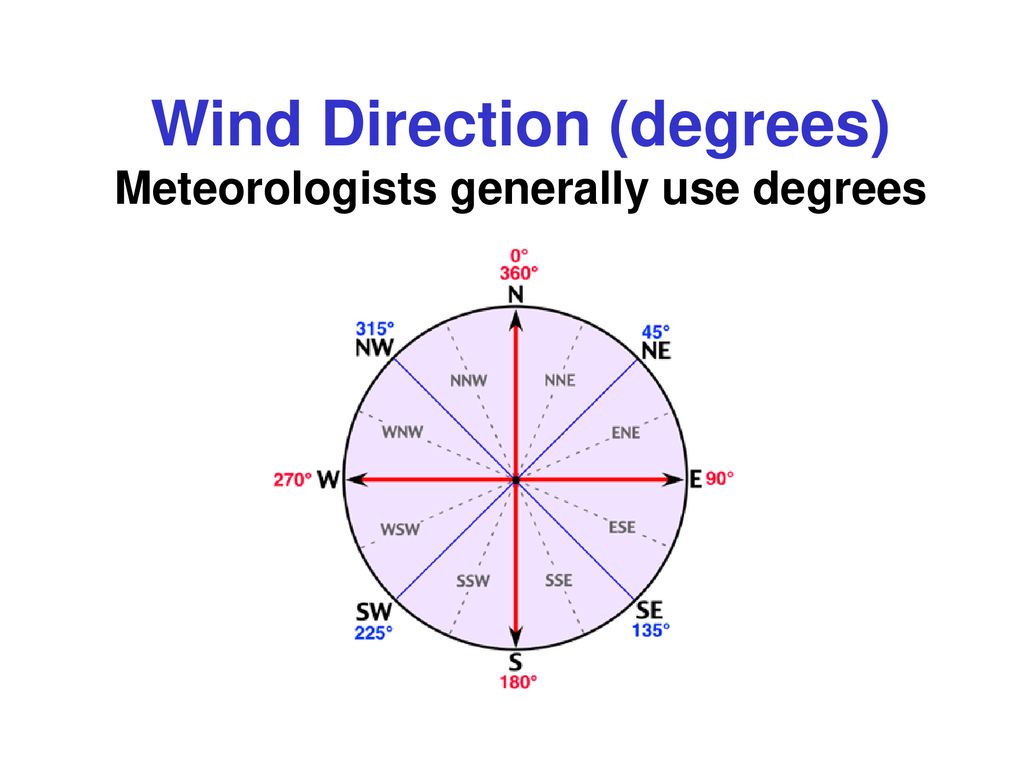
A) Sometimes wind directions are conveyed as greater than 360 degrees. Numbers above 360 indicate a second loop around the quadrant. For example:
450 degrees is the same as 90 degrees
720 degrees is the same as 0 degrees
Given wind directions that are greater than 360, write some code to return the wind direction expressed between 0 and 360. Check your code works against both of the numbers below.
large_wind_direction = 512
large_wind_direction = 750
large_wind_direction % 360
30
Hint check out the Python arithmetic operators
B) Write a statement to check if a given wind direction is coming from the west.
wind_direction = 145
# Due west only
wind_direction == 270
False
# Range of degrees
wind_direction > 240 and wind_direction < 300
False
C) Write a block of code that takes a wind direction and prints back to the user the direction the wind is coming from. You can use a range of values for each direction (i.e. “east” means wind coming from between 45 and 135 degrees).
wind_direction = 132
if wind_direction > 45 and wind_direction <= 135:
print('East')
elif wind_direction > 135 and wind_direction <= 225:
print('South')
elif wind_direction > 225 and wind_direction <= 315:
print('West')
elif (wind_direction > 315 and wind_direction <= 360) or wind_direction < 45:
print('North')
else:
print('invalid wind direction')
East
C) Monsoon winds in some regions are identified by winds coming from the Northwest (+/- 30 degrees) at greater than or equal to 20 mph. Write a statement to evaluate if the wind direction/wind speed combination would qualify as a monsoon-type wind.
wind_speed = 22
wind_direction = 43
if wind_speed > 20 and wind_direction > 285 and wind_direction < 345:
print('monsoon wind')
else:
print('not a monsoon wind')
not a monsoon wind
Question 6#
Given a temperature in fahrenheit, write an equation to convert the temperature to celsius. Print out a statement after converting to celsius informing the user whether or not the temperature has reached boiling point of water.
fahrenheit = 87
celsius = (fahrenheit - 32)*5/9
print(celsius)
30.555555555555557